While custom forms allow you to customize the requests, there are scenarios requiring you to use a programatic approach when handling requests. For example, when Automation Orchestrator is used for external integrations requiring triggering requests or when automating requests.
In vRealize Automation 7.x, you can manage requests with the VMware Aria Automation plug-in or matching REST API:
From a catalog item get the provisioning request data by using
getProvisioningRequestForCatalogItem()
andgetProvisioningRequestData()
. The provisioning request data is a type of template similar to the VMware Aria Automation 8.x cloud template YAML, but here formatted in JSON.Update the provisioning request data.
Use
requestCatalogItemWithProvisioningRequest(catalogItem, provisioningRequest)
.
This provisioning request data is a complex object including many fields that are not necessarily matching what the end user would see at request time. For example to change the number of CPUs it is necessary to change provisioningRequestData.ComponentName.data.cpu = cpuNb
. It is also mandatory to set some fields like the business group ID, which is the equivalent of a VMware Aria Automation 8.x project.
In VMware Aria Automation 8.x, requesting a catalog item programmatically is simpler. The request is done by using the Service Broker API /catalog/api/items/{id}/request
. The body of the request includes:
deploymentName
projectId
requestCount
The request inputs defined in the YAML.
The requests returns an array of deployment IDs since some cloud templates support more than 1 request.
The following is an example of a request body:
{ "deploymentName": "TestRequest", "projectId": "1628469a-3f98-44f1-ba80-e9ee610686a3", "bulkRequestCount": 1, "inputs": { "platform": "platform:vsphere", "environment": "environment:production" } }
The input keys can be obtained with a GET /catalog/api/items/" + catalogItemId
call. The sample action getCatalogItemInputProperties
does that and outputs the list of inputs in a data grid.
The following example includes sample code from the createCatalogItemRequest
action:
var url = "/catalog/api/items/" + catalogItemId + "/request"; var requestBody = { "deploymentName": deploymentName, "projectId": projectId, "bulkRequestCount": bulkRequestCount, "inputs": inputProperties } var content = JSON.stringify(requestBody); var operation = "POST"; try { var contentAsString = System.getModule("com.vmware.vra.extensibility.plugin.rest").invokeRestOperation(vraHost, operation, url, content); } catch (e) { throw "POST " + url + "Failed" + "\n Error : " + e; } var deployments = JSON.parse(contentAsString); var deploymentsIds = new Array(); for each (var deployment in deployments) { deploymentsIds.push(deployment.deploymentId); } return deploymentsIds;
The sample workflow Request Catalog Item (Service Broker Only)
lists the catalog items in a drop-down menu. The list of inputs is preconfigured so the value can be edited and when submitted, run the createCatalogItemRequest
action.
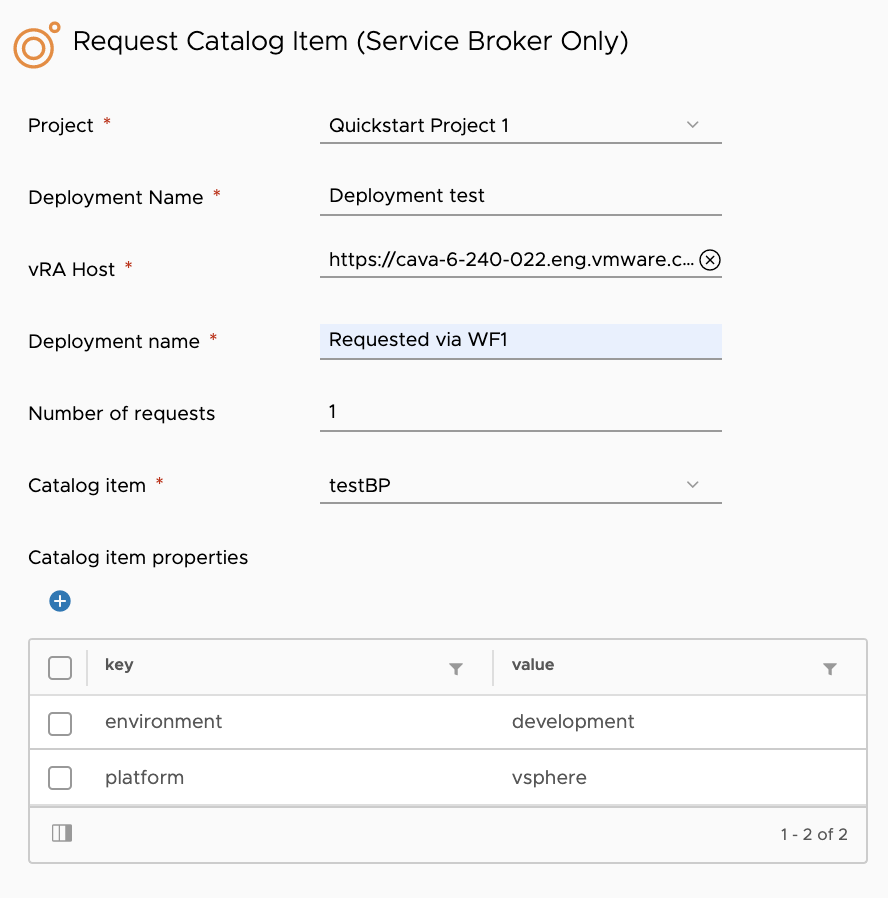