As a cloud template designer, you use input parameters so that users can make custom selections at request time.
How inputs work
When users supply inputs, you no longer need to save multiple copies of templates that are only slightly different. In addition, inputs can prepare a template for day 2 operations. See How to use cloud template inputs for vRealize Automation day 2 updates.
The following inputs show how you might create one cloud template for a MySQL database server, where users can deploy that one template to different cloud resource environments and apply different capacity and credentials each time.
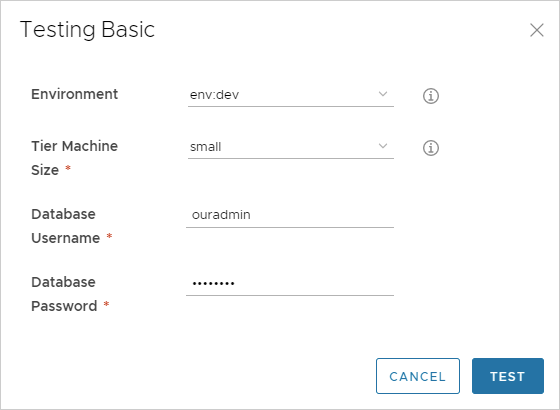
Adding input parameters
Add an inputs
section to your template code, where you set the selectable values.
In the following example, machine size, operating system, and number of clustered servers are selectable.
inputs: wp-size: type: string enum: - small - medium description: Size of Nodes title: Node Size wp-image: type: string enum: - coreos - ubuntu title: Select Image/OS wp-count: type: integer default: 2 maximum: 5 minimum: 2 title: Wordpress Cluster Size description: Wordpress Cluster Size (Number of nodes)
If you're uncomfortable editing code, you can click the code editor Inputs tab, and enter settings there. The following example shows some inputs for the MySQL database mentioned earlier.
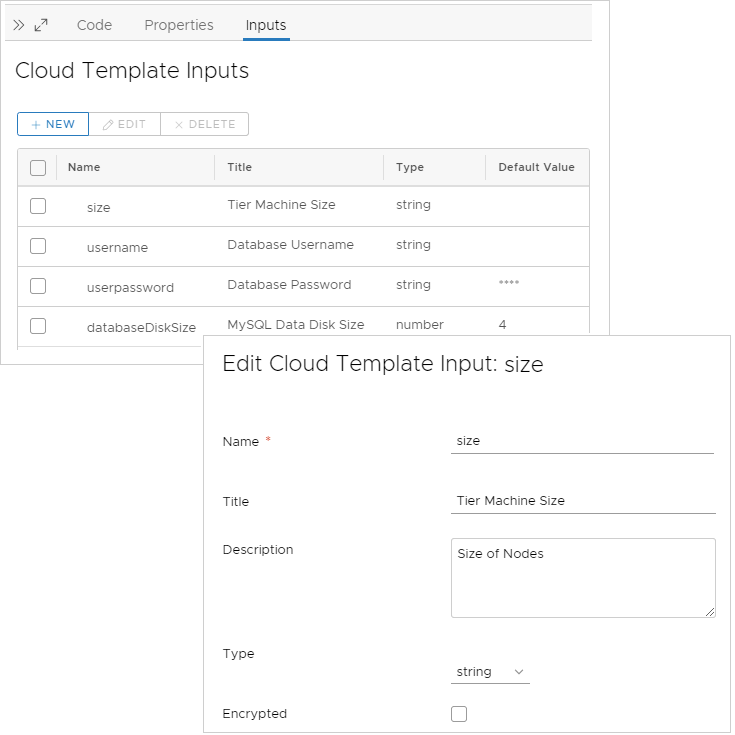
Referencing input parameters
Next, in the resources
section, you reference an input parameter using ${input.property-name}
syntax.
If a property name includes a space, delimit with square brackets and double quotes instead of using dot notation: ${input["property name"]}
input
except to indicate an input parameter.
resources: WebTier: type: Cloud.Machine properties: name: wordpress flavor: '${input.wp-size}' image: '${input.wp-image}' count: '${input.wp-count}'
Nested input
If you want to organize or categorize inputs, nested input is supported. In the following example, the CPU and memory are together under a parent heading of level.
inputs: cluster: type: integer title: Cluster default: 1 minimum: 1 maximum: 4 level: type: object properties: cpu: type: integer title: CPU default: 1 minimum: 1 maximum: 4 memory: type: integer title: Memory default: 2048 minimum: 2048 maximum: 4096
In the resources section, to reference the nested input, include the parent in the path.
resources: Disk_1: type: Cloud.vSphere.Disk allocatePerInstance: true properties: capacityGb: 1 count: ${input.cluster} Machine_1: type: Cloud.vSphere.Machine allocatePerInstance: true properties: totalMemoryMB: ${input.level.memory} attachedDisks: - source: ${slice(resource.Disk_1[*].id, count.index, count.index + 1)[0]} count: ${input.cluster} imageRef: ubuntu cpuCount: ${input.level.cpu}
Optional versus required input
For all types except Boolean, user entry is optional by default. To require input, do one of the following:
- Set a default value.
- When you have no nested inputs, add the
populateRequiredOnNonDefaultProperties
property:inputs: cluster: type: integer populateRequiredOnNonDefaultProperties: true title: Cluster minimum: 1 maximum: 4
Note that you can also apply this setting when referencing a formal property group:
inputs: pgmachine: type: object populateRequiredOnNonDefaultProperties: true $ref: /ref/property-groups/machine
- With nested inputs, add the
populateRequiredForNestedProperties
property:inputs: cluster: type: integer title: Cluster default: 1 minimum: 1 maximum: 4 level: type: object properties: cpu: type: integer populateRequiredForNestedProperties: true title: CPU minimum: 1 maximum: 4 memory: type: integer populateRequiredForNestedProperties: true title: Memory minimum: 2048 maximum: 4096
Note that you can also apply this setting when a formal property group reference is nested:
level: type: object properties: cpu: type: integer populateRequiredForNestedProperties: true title: CPU minimum: 1 maximum: 4 memory: type: integer populateRequiredForNestedProperties: true title: Memory minimum: 2048 maximum: 4096 pgrequester: type: object populateRequiredForNestedProperties: true $ref: /ref/property-groups/requesterDetails
Optional input—To force input to remain optional, set an empty default value using tick marks:
owner: type: string minLength: 0 maxLength: 30 title: Owner Name description: Account Owner default: ''
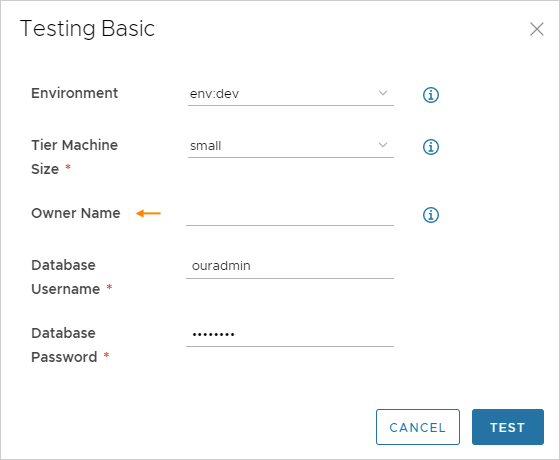
List of input properties
Property | Description |
---|---|
const | Used with oneOf. The real value associated with the friendly title. |
default | Prepopulated value for the input. The default must be of the correct type. Do not enter a word as the default for an integer. |
description | User help text for the input. |
encrypted | Whether to encrypt the input that the user enters, true or false. Passwords are usually encrypted. You can also create encrypted properties that are reusable across multiple cloud templates. See Secret Cloud Assembly properties. |
enum | A drop-down menu of allowed values. Use the following example as a format guide. enum: - value 1 - value 2 |
format | Sets the expected format for the input. For example, (25/04/19) supports date-time. Allows the use of the date picker in Service Broker custom forms. |
items | Declares items within an array. Supports number, integer, string, Boolean, or object. |
maxItems | Maximum number of selectable items within an array. |
maxLength | Maximum number of characters allowed for a string. For example, to limit a field to 25 characters, enter |
maximum | Largest allowed value for a number or integer. |
minItems | Minimum number of selectable items within an array. |
minLength | Minimum number of characters allowed for a string. |
minimum | Smallest allowed value for a number or integer. |
oneOf | Allows the user input form to display a friendly name (title) for a less friendly value (const). If setting a default value, set the const, not the title. Valid for use with types string, integer, and number. |
pattern | Allowable characters for string inputs, in regular expression syntax. For example, |
properties | Declares the key:value properties block for objects. |
readOnly | Used to provide a form label only. |
title | Used with oneOf. The friendly name for a const value. The title appears on the user input form at deployment time. |
type | Data type of number, integer, string, Boolean, or object.
Important:
A Boolean type adds a blank checkbox to the request form. Leaving the box untouched does not make the input False. To set the input to False, users must check and then clear the box. |
writeOnly | Hides keystrokes behind asterisks in the form. Cannot be used with enum. Appears as a password field in Service Broker custom forms. |
Additional examples
String with enumeration
image: type: string title: Operating System description: The operating system version to use. enum: - ubuntu 16.04 - ubuntu 18.04 default: ubuntu 16.04 shell: type: string title: Default shell Description: The default shell that will be configured for the created user. enum: - /bin/bash - /bin/sh
Integer with minimum and maximum
count: type: integer title: Machine Count description: The number of machines that you want to deploy. maximum: 5 minimum: 1 default: 1
Array of objects
tags: type: array title: Tags description: Tags that you want applied to the machines. items: type: object properties: key: type: string title: Key value: type: string title: Value
String with friendly names
platform: type: string oneOf: - title: AWS const: platform:aws - title: Azure const: platform:azure - title: vSphere const: platform:vsphere default: platform:aws
String with pattern validation
username: type: string title: Username description: The name for the user that will be created when the machine is provisioned. pattern: ^[a-zA-Z]+$
String as password
password: type: string title: Password description: The initial password that will be required to logon to the machine. Configured to reset on first login. encrypted: true writeOnly: true
String as text area
ssh_public_key: type: string title: SSH public key maxLength: 256
Boolean
public_ip: type: boolean title: Assign public IP address description: Choose whether your machine should be internet facing. default: false
Date and time calendar selector
leaseDate: type: string title: Lease Date format: date-time