Using extensibility actions you can integrate Automation Assembler with an Enterprise ITSM, like ServiceNow.
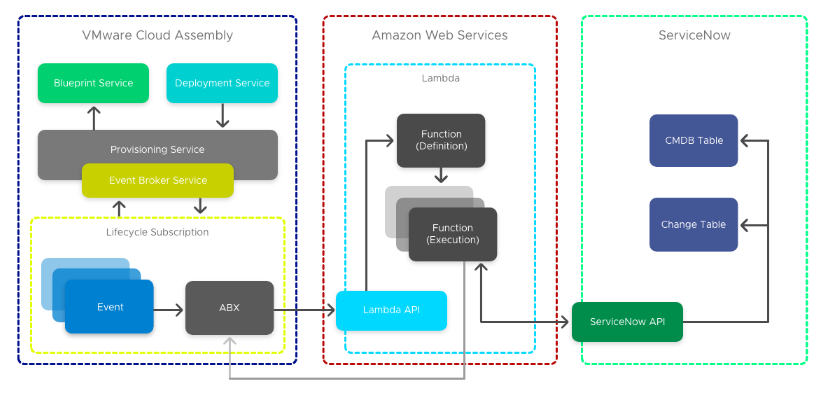
To create this integration, you use four extensibility action scripts. The first three scripts are initiated in sequence during provisioning, at the compute provision post event. The fourth script triggers at the compute removal post event.
For more information on event topics, refer to Event topics provided with Automation Assembler.
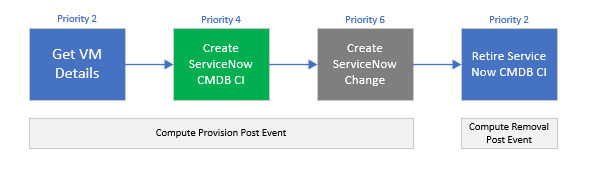
Get VM Details
The Get VM details script acquires additional payload details required for CI creation and an identity token that is stored in Amazon Web Services Systems Manager Parameter Store (SSM). Also, this script updates customProperties with additional properties for later use.
Create ServiceNow CMDB CICreate ServiceNow Change
This script finishes the ITSM integration by passing the ServiceNow instance URL as an input and storing the ServiceNow credentials as SSM to meet security requirements.
Create ServiceNow Change
The retire ServiceNow CMDB CI script prompts the ServiceNow to stop and marks the CI as retired based on the custom property serviceNowSysId that was created in the creation script.
Prerequisites
- Before configuring this integration, filter all event subscriptions with the conditional cloud template property: event.data["customProperties"]["enable_servicenow"] === "true"
Note: This property exists on cloud templates that require a ServiceNow integration.
- Download and install Python.
For more information on filtering subscriptions, see .Create an extensibility subscription.
Procedure
Results
Automation Assembler is successfully integrated with ITSM ServiceNow.
What to do next
from botocore.vendored import requests import json import boto3 client = boto3.client('ssm','ap-southeast-2') def handler(context, inputs): snowUser = client.get_parameter(Name="serviceNowUserName",WithDecryption=False) snowPass = client.get_parameter(Name="serviceNowPassword",WithDecryption=True) tableName = "cmdb_ci_vmware_instance" sys_id =inputs['customProperties']['serviceNowSysId'] url = "https://" + inputs['instanceUrl'] + "/api/now/"+tableName+"/{0}".format(sys_id) headers = {'Content-type': 'application/json', 'Accept': 'application/json'} payload = { 'state': 'Retired' } results = requests.put( url, json=payload, headers=headers, auth=(inputs['username'], inputs['password']) ) print(results.text)